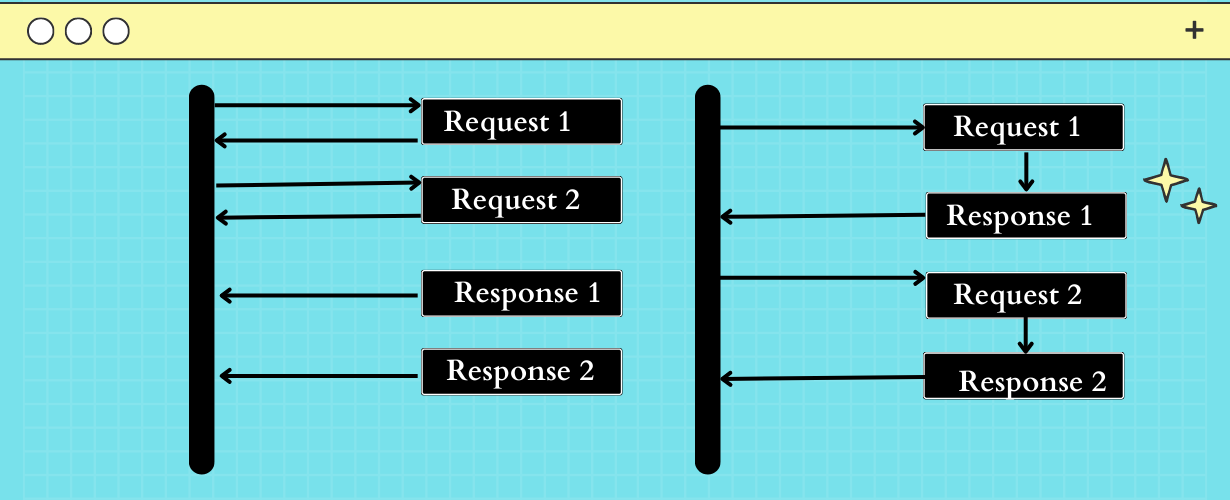
01
How To Send Asynchronous Requests with Guzzle HTTP Client
Guzzle is a popular PHP HTTP client library that allows you to send HTTP requests and interact with web services easily. It supports asynchronous requests, which allows you to send multiple requests concurrently, improving the overall performance of your application. In this example, we'll show you how to make asynchronous requests using Guzzle's asynchronous functionality. And we'll Compare it with synchronous request.
1. Installing Guzzle
First, you need to make sure you have Guzzle installed in your project. Or You can do this using Composer.I will be using Laravel for this code example however the proccess is the same for all php frameworks:
composer require guzzlehttp/guzzle
2. Using Guzzle to Make Asynchronous Requests
Now, let's see how to use Guzzle to send asynchronous requests. we'll create an API endpoint that takes 5 seconds to response.
Route::get('slow-url',function (){
//Each request takes 5 seconds
$time = 5;
sleep($time);
return response()->json([
'time' => $time,
'message' => 'success'
]);
});
- We'll start the execution by saving current in
$time_start
variable. - We first import the required classes using the use statement.
- We create a new Guzzle HTTP client using
$client = new Client();.
- We define an array of promises named
$promises
, where each promise represents an asynchronous request. In this example, we have five GET requests tohttp://example.test/api/slow-url
. You can add more requests to the$promises
array as needed. - After creating the promises, we use
Promise\Utils::unwrap($promises)
to wait for all the promises to complete. This line will block execution until all the requests are finished. - The results of the requests are now available in the
$results
array, where each key corresponds to the key used in the$promises
array. - Finally, we can access the response bodies of each request using the
$results
array , dump their content and total execution time.
$time_start = microtime(true);
// Create a new Guzzle HTTP client
$client = new GuzzleHttp\Client();
// Create an array of promises for each async request
$promises = [
'request1' => $client->getAsync(url('api/slow-url')),
'request2' => $client->getAsync(url('api/slow-url')),
'request3' => $client->getAsync(url('api/slow-url')),
'request4' => $client->getAsync(url('api/slow-url')),
'request5' => $client->getAsync(url('api/slow-url')),
// Add more async requests as needed
];
// Wait for all promises to complete
$results = GuzzleHttp\Promise\Utils::unwrap($promises);
// Access the response of each request
dump((string)$results['request1']->getBody() );
dump((string)$results['request2']->getBody());
dump((string)$results['request3']->getBody());
dump((string)$results['request4']->getBody());
dump((string)$results['request5']->getBody());
$time_end = microtime(true);
$execution_time = ($time_end - $time_start);
dd("Total Execution Time of async-request: {$execution_time} seconds");
The Total Execution time as we can see in the follwoing image is around 5 seconds. In case of synchronous it could be No. of requests x API response time
ie 25 seconds.
Conlusion:
Using Guzzle's asynchronous requests can significantly improve the performance of your application, especially when dealing with multiple external services or APIs. Asynchronous requests allow you to send multiple requests concurrently, saving time and resources. However, be mindful of not overwhelming the server with too many concurrent requests and consider implementing error handling and proper timeouts for better reliability.
Also Read: How to Send Asynchronous Requests with cURL in PHP